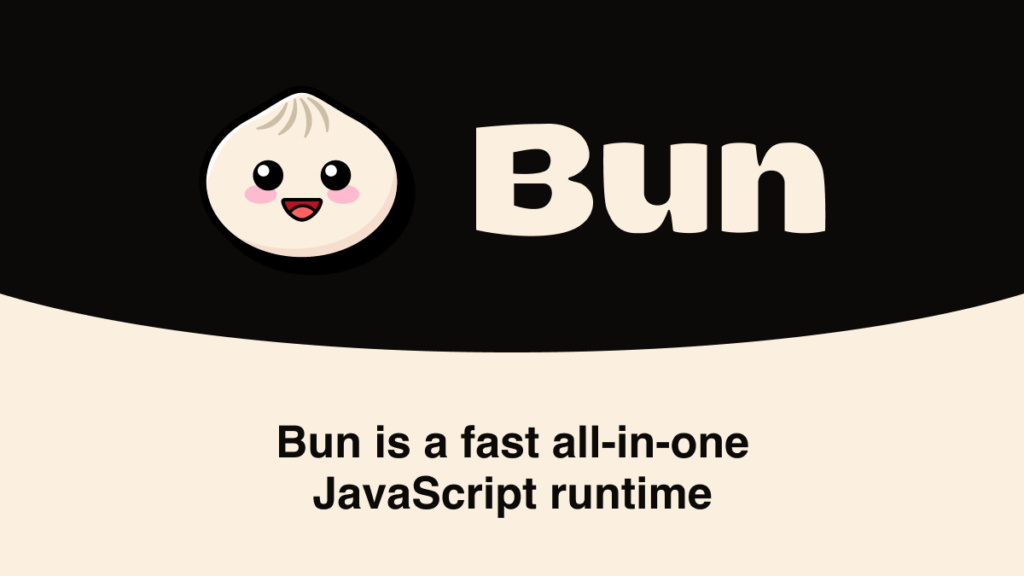
Bun.js is a new JavaScript runtime designed to be fast and efficient. It can build various applications, including web servers, APIs, and CLI tools.
Express.js is a popular Node.js framework for building web applications and APIs. It provides a simple, elegant way to define routes, handle requests, and render responses.
This article will show you how to write Express-like APIs with Bun.js.
Getting started
To get started, you must install Bun.js and the Bunrest package. Bun.js can be installed using the following command(Linux or Windows subsystem Linux required):
curl -fsSL https://bun.sh/install | bash
The bunrest package can be installed using the following command:
npm i bunrest
Creating a server
We can use the App class from the bunrest package to create a server. The following code shows how to create a simple server:
import App from 'bunrest'
const app = App();
Adding middlewares
Middleware is a function that can be used to pre-process or post-process requests. Express.js provides built-in middleware, such as logging, authentication, and compression.
We can use the use() method to add middleware to our server. The following code shows how to add a middleware that logs all requests:
app.use((req, res, next) => {
console.log('Request Log:', req.method, req.path);
next();
});
Adding a router
The router is responsible for matching requests to routes and handling them accordingly. Bunrest provides a simple router that can be used to define routes and handlers.
The following code shows how to define a route that handles GET requests to the /
path:
app.get('/', (req, res) => {
res.send('I am Bun!');
});
Starting the server
To start the server, we can use the listen() method. The following code shows how to create the server on port 3000:
app.listen(3000, () => {
console.log('App is runing on port 3000');
});
Conclusion
In this article, we have shown you how to write Express-like APIs with Bun.js. Bunrest is a simple and easy-to-use package that can be used to create powerful and efficient APIs.
Bunrest is a powerful and efficient tool for building Express-like APIs with Bun.js. It is easy to use and provides several features, making it an excellent choice for all developers.
Subscribe to our email newsletter to get the latest posts delivered right to your email.
Comments